Welcome back, At the end of the last tutorial i wondered what would happen if we had a platform that you could jump underneath from.
Saturday, 29 March 2008
creating a platform game in as3 part 5
Friday, 28 March 2008
creating a platform game in as3 part 4
In the end of the last post we had a problem where the character vibrated. This was because when ever the character touched the floor i made it move up by one pixel. to rectify this i will make another hittest that detects when the character is one pixel off the floor and only calculate the velocity of the player if it returns false.
function run(event:Event) {
velocity+=gravity;
if (land.hitTestPoint(char.x, char.y+char.height/2, true)) {
velocity = 0;
char.y--
}
if (!land.hitTestPoint(char.x, char.y+char.height/2+1, true)) {
char.y += velocity;
} else {
velocity=0;
if (attemptJump==true) {
char.y--;
velocity =-5;
}
}
if (goleft==true) {
char.x+=lspeed;
}
if (goright==true) {
char.x+=rspeed;
}
}
function run(event:Event) {
velocity+=gravity;
if (land.hitTestPoint(char.x, char.y+char.height/2, true)) {
velocity = 0;
char.y--;
}
if (!land.hitTestPoint(char.x, char.y+char.height/2+1, true)) {
char.y += velocity;
} else {
velocity=0;
if (attemptJump==true) {
char.y--;
velocity =-5;
}
}
if (!land.hitTestPoint(char.x-char.width/2, char.y+char.height/4, true)) {
if (goleft==true) {
char.x+=lspeed;
}
}
if (!land.hitTestPoint(char.x+char.width/2, char.y+char.height/4, true)) {
if (goright==true) {
char.x+=rspeed;
}
}
}
creating a platform game in as3 part 3
import flash.display.*;
import flash.events.*;
stage.frameRate = 30;
stage.addEventListener(Event.ENTER_FRAME, run);
stage.addEventListener(KeyboardEvent.KEY_DOWN, keyPressed);
stage.addEventListener(KeyboardEvent.KEY_UP, keyReleased);
var gravity:Number = 0.2;
var velocity:Number = 0;
var lspeed:int = -2;
var rspeed:int = 2;
var goleft:Boolean = false;
var goright:Boolean=false;
var attemptJump:Boolean=false;
var char:MovieClip = new character();
char.x=200;
char.y=0;
stage.addChild(char);
var land:MovieClip = new ground();
land.x=200;
land.y=300;
stage.addChild(land);
function keyPressed(event:KeyboardEvent):void {
switch (event.keyCode) {
case Keyboard.UP :
attemptJump = true;
break;
case Keyboard.LEFT :
goleft = true;
break;
case Keyboard.RIGHT :
goright = true;
break;
}
}
function keyReleased(event:KeyboardEvent):void {
switch (event.keyCode) {
case Keyboard.UP :
attemptJump = false;
break;
case Keyboard.LEFT :
goleft = false;
break;
case Keyboard.RIGHT :
goright = false;
break;
}
}
function run(event:Event) {
velocity+=gravity;
if (land.hitTestPoint(char.x, char.y+char.height/2, true)) {
velocity = 0;
char.y--;
if (attemptJump==true) {
velocity =-5;
}
} else {
char.y += velocity;
}
if (goleft==true) {
char.x+=lspeed;
}
if (goright==true) {
char.x+=rspeed;
}
}
Thursday, 27 March 2008
creating a platform game in as3 part 2
Read creating a platform game in as3 if you haven't already.
import flash.events.*;
stage.frameRate = 30;
stage.addEventListener(Event.ENTER_FRAME, run);
stage.addEventListener(KeyboardEvent.KEY_DOWN, keyPressed);
stage.addEventListener(KeyboardEvent.KEY_UP, keyReleased);
var gravity:Number = 0.2;
var velocity:Number = 0;
var lspeed:int = -2;
var rspeed:int = 2;
var goleft:Boolean = false;
var goright:Boolean=false;
var attemptJump:Boolean=false;
var char:MovieClip = new MovieClip();
char.graphics.lineStyle(2, 0x112233);
char.graphics.beginFill(0x112233);
char.graphics.drawRect(200, 0, 20, 50);
char.graphics.endFill();
addChild(char);
var land:MovieClip = new MovieClip();
land.graphics.lineStyle(2, 0x112233);
land.graphics.beginFill(0x112233);
land.graphics.drawRect(0, 350, 400, 25);
land.graphics.endFill();
addChild(land);
function keyPressed(event:KeyboardEvent):void {
switch (event.keyCode) {
case Keyboard.UP :
attemptJump = true;
break;
case Keyboard.LEFT :
goleft = true;
break;
case Keyboard.RIGHT :
goright = true;
break;
}
}
function keyReleased(event:KeyboardEvent):void {
switch (event.keyCode) {
case Keyboard.UP :
attemptJump = false;
break;
case Keyboard.LEFT :
goleft = false;
break;
case Keyboard.RIGHT :
goright = false;
break;
}
}
function run(event:Event) {
char.y+=velocity;
velocity+=gravity;
if (char.hitTestObject(land)) {
velocity = 0;
}
if (attemptJump==true) {
velocity =-5;
}
if (goleft==true) {
char.x+=lspeed;
}
if (goright==true) {
char.x+=rspeed;
}
}
function run(event:Event) {
char.y+=velocity;
velocity+=gravity;
if (char.hitTestObject(land)) {
velocity = 0;
if (attemptJump==true) {
velocity =-5;
}
}
if (goleft==true) {
char.x+=lspeed;
}
if (goright==true) {
char.x+=rspeed;
}
}
Creating a platform game in as3
I've always wanted to create a platform game, so here goes.
import flash.display.*;
import flash.events.*;
stage.frameRate = 30;
stage.addEventListener(Event.ENTER_FRAME, run);
var gravity:Number = 0.2;
var velocity:Number = 0;
var char:MovieClip = new MovieClip();
char.graphics.lineStyle(2, 0x112233);
char.graphics.beginFill(0x112233);
char.graphics.drawRect(275, 0, 20, 50);
char.graphics.endFill();
addChild(char);
var land:MovieClip = new MovieClip();
land.graphics.lineStyle(2, 0x112233);
land.graphics.beginFill(0x112233);
land.graphics.drawRect(0, 350, 550, 25);
land.graphics.endFill();
addChild(land);
function run(event:Event) {
if (char.hitTestObject(land)) {
velocity = 0;
}
char.y+=velocity;
velocity+=gravity;
}
Tuesday, 25 March 2008
Controlling timeline events
In Flash, if you want to stop, play or move to a certain frame in the movie you have to use actionscript.
First - stop. To stop a flash movie you simply have to write stop() in the actions panel of the frame you want the movie to stop at.
playbut.addEventListener(MouseEvent.MOUSE_DOWN, playFunction);
stopbut.addEventListener(MouseEvent.MOUSE_DOWN, stopFunction);
nextframebut.addEventListener(MouseEvent.MOUSE_DOWN, nextframeFunction);
prevframebut.addEventListener(MouseEvent.MOUSE_DOWN, prevframeFunction);
function playFunction(Event:MouseEvent):void {
play()
}
function stopFunction(Event:MouseEvent):void {
stop()
}
function nextframeFunction(Event:MouseEvent):void {
nextFrame()
}
function prevframeFunction(Event:MouseEvent):void {
prevFrame()
}
Buttons in as3
The first thing i did when i opened CS3 for the first time was tried to create a button.
- Open up a new Flash Actionscript 3 file in the way that i explained before.
- Draw a button shape.
- Highlight the button shape and press F8. A box should pop up that looks like this.
- Click on "Button" in the type section and name the button appropriately.
- Press "OK" to continue, which should take you back to the stage where you're button is.
- Click on your button and look to the bottom of the screen, where the properties bar should be.
- Write an instance name in the box provided. I have called mine "buttonTest". This is important for when we do the script.
- Now we are ready to write the Actionscript for this button. All the button is going to do is trace a message in the output panel. Make a new layer and call in "actions". To do this click on the "insert layer" button, where my cursor is and then double click on the new layer title, "Layer 2" to edit it's name.
- Click on the frame head of layer 2 frame one, which should be a white rectangle with a white circle in the middle, like the one in this image, and press alt+F9 to open the action panel.
- This is where we have to start coding the button. In the actions panel that you just opened write...
import flash.events.MouseEvent;
buttonTest.addEventListener(MouseEvent.MOUSE_DOWN, traceFunction);
function tracefunction(Event:MouseEvent):void{
trace("Button Clicked")
}
line 1 - importing the Mouse Events
line 2 I'm adding event listeners to the buttons instance names that i have. When the mouse is touching buttonTest and it is pressed, do the traceFunction.
line 3-5 This is the traceFunction. It is a MouseEvent function that is executed when the buttonTest is pressed. When it is pressed the trace function is called which traces the message "Button Clicked" in the output panel.
- Test your movie by pressing Command+Enter, (control+Enter on windows)
- You should see that when you click the button a message comes up in the output panel that says "Button Clicked".
- Thats all for the button tutorial, i will explain how to make functions in a later post.
Getting started with as3
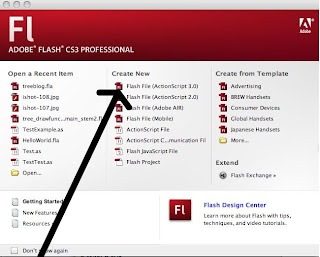
Welcome
Well, as of now i have a blog! Which i will dedicate to helping you (and me) learn as3.